Arduino store values using EEPROM memory
EEPROM (Electrically erasable programmable read-only memory) is a non-volatile memory in which the individual segments can be erased and reprogrammed by using electric signals. EEPROM memory can permanently store the data which can retain after the power off. EEPROM memories are said to have a data retention span of more than 10 years.
Arduino EEPROM memory
The main use of the EEPROM memories are for applications like last settings reminder, last value save, last state backup, etc, where the data need to be saved and to be retrieved on the next power on. In an Arduino sketch, if a generated value is stored in a variable, it remains only as long as the power is not interrupted. If the Arduino resets or the power is interrupted the data stored will disappear and it initializes with the default value declared in the sketch.
In devices that often repeat the last settings and for inputs that are rarely changed like changeable passwords, pins, etc, EEPROM memory is a suitable option.
The EEPROM memory will not be erased while uploading a new sketch or resetting the arduino, a data stored to an EEPROM address can be only erased by an EEPROM write operation.
Arduino microcontroller boards have different EEPROM sizes like 512KB, 1KB, 4KB, etc. Common Arduino boards that have ATMEGA328P microcontrollers which has an EEPROM storage space of 1KB or 1024 bytes.
A single byte is 8 bits of information. Each memory location of the EEPROM can store only 1 byte, that is 8-bit numbers. An 8 bit can store numbers from 0 to 255 (00000000 to 11111111); 2^8 total 256 values. So, a microcontroller with 1KB EEPROM memory can store 8 bits of information in each address from 0 to 1023.
EEPROM is specified to handle 100 000 writes or erase cycles, so every time when a write or erase operation is performed at an EEPROM address its life expectancy is reducing by one. So loops that repeatedly write EEPROM value or operations that write or erase very often should be avoided to save the EEPROM life. Repeated operation in the same address location reduces the life of the particular address, so it is better to use the EEPROM addresses evenly distributed. But reading the EEPROM memory is unlimited as it doesn’t involve any write/erase operation.
Arduino has an inbuilt EEPROM library that provides functions to easily write and read its EEPROM memory.
Write
To store data to an address,
EEPROM.write(address, data);
Writing again to the same address replace the existing value with a new value.
Read
To read data from an address.
data = EEPROM.read(address);
Update
EEPROM.update(address, data);
The update function is the same as the write function. But an update function only writes the value if the new value is not the same as the existing value.
An equivalent code for the update function is given below, the EEPROM writes only if the existing data at the specified address is not equal to the new data.
if( EEPROM.read(address) != data){ EEPROM.write(address, data); }
The update function helps to avoid wearing out EEPROM from writing the same data again.
EEPROM write and read using Arduino serial monitor
In this code, the EEPROM memory can be written and read through the Arduino serial monitor.
Code
#include <EEPROM.h> int address = 0, number = 0; char command; void setup() { Serial.begin(9600); } void loop() { while (Serial.available() > 0) { command = Serial.read(); address = Serial.parseInt(); number = Serial.parseInt(); } switch (command) { case 'w' : // writes value to address. EEPROM.write(address, number); Serial.print("Write Address "); Serial.print(address); Serial.print(" <-- "); Serial.println(number); command = '0'; break; case 'r' : // read address. number = EEPROM.read(address); Serial.print("Read Address "); Serial.print(address); Serial.print(" --> "); Serial.println(number); command = '0'; break; } delay(50); }
Here to write a value to a particular EEPROM address enter w,0,200 (the value 200 will be stored to address 0; ‘w’ indicates as write).
Like above enter r,0 (r indicates to read, and the value followed by a comma is the address of the EEPROM memory).
This is code is useful for store and read the EEPROM memory with random addresses and data.
What happens if the integer value of the input data is above 255 in EEPROM.write?
The Arduino stores the data in the specified address as an 8-bit binary number. If the data is above 255 it stores only the 8 bits of the value starting from the LSB (Least significant bit).
For example, if the input number is 300, the value stored in the EEPROM address will be 00101100 which is integer 44.
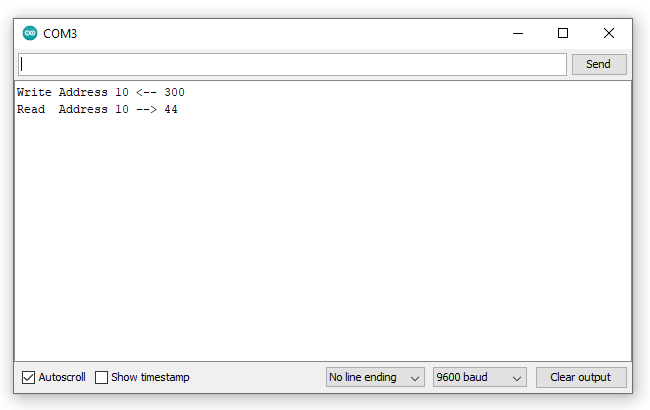
Write integer 300 to address 10 and read address 10
Because the binary of 300 is 100101100 which saves only the 8 bits which eliminates the MSB 1.
9 bits binary, 1 0010 1100 –> 300,
8 bits from LSB, 0010 1100 –> 44
What happens if the address of the EEPROM.write function is above 1023?
Similar to the above case, the address is a 10-bit binary number. Hence it takes only 10 bits from the LSB side and eliminates the bits after the 10th bit.
For example, use an Address as 1500 which has a binary value 10111011100.
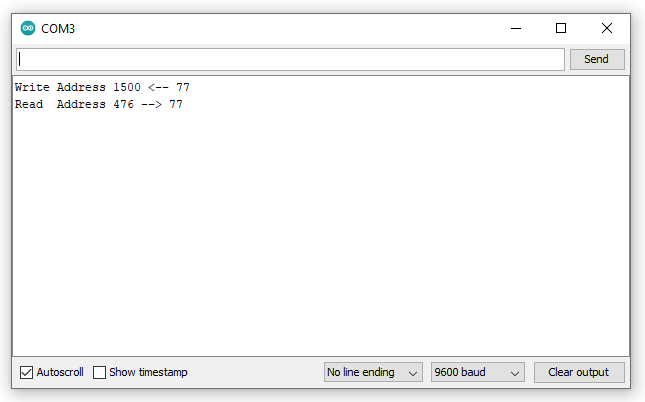
Write integer 77 to address 1500 and read address 476
Only 10 bits will be taken from LSB which is 0111011100, the resultant integer value is 476, so the data will be stored to the address 476.
See how much difference it has made, the address entered was 1500 but the data is stored in 476. These types of mistakes should be avoided else the value might be stored at the wrong locations or overwrite the data at some other location.